Modern problems require modern solutions. And the need for fast performance has always been a problem. NCache is a quick fix for your critical issues, such as performance and scalability. It works efficiently and covers all the problematic areas in your application, although caching has its primary purpose for more read-intensive applications. This blog thoroughly explains how NCache is the best choice for your read-intensive applications.
NCache Details Scalability in NCache NCache Performance
The Need for Caching
Let’s start with the beginning and see why caching is needed? Its simple answer is databases’ response time. It is a given that the primary storage (your database) containing all the data in one place can choke due to a higher response rate. What solves it? Caching – as it provides in-memory storage and a faster response time.
Secondly, unlike a database, a cache being distributed in nature has no single point of failure, The load is divided and thus fewer chances of requests making the servers choke.
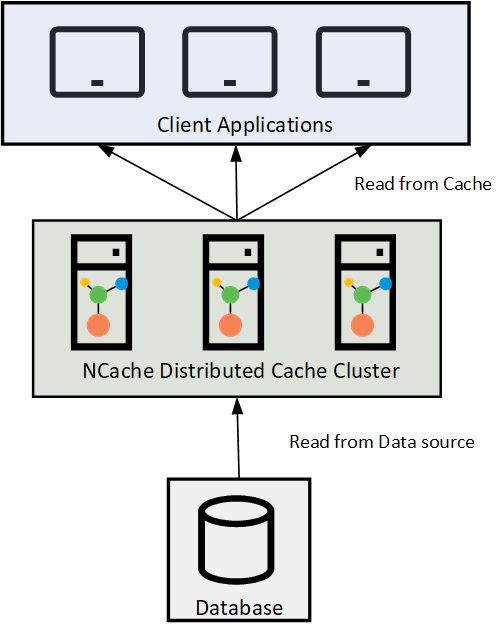
Figure 1: Read Intensive Applications with NCache
Given the above facts we’ve just discussed, we have established that caching is the best fit for situations where your write intensity is not very high, but the read intensity is relatively higher.
Let us consider a website containing information about the transport system. The bus fares and the timings are usually static, meaning not changed frequently. Such applications with more read-intensive data have a rich set of features provided by NCache for performing faster read operations.
NCache Details NCache For Data Caching High Availability
NCache as a Read Intensive Cache Store
Applications with a read intensive nature, have NCache to perform all the read operations as fast as possible. NCache has a rich set of features catering all kinds of read operations like fetching single or bulk items. Once the cache receives data from the database, all the fetch operations go directly to the cache for higher performance and scalability.
NCache Details CRUD Operations Scalability in NCache
Get Operations in NCache
NCache provides various overloads of the Get() method to fetch data from the cache against a specific key. These operations are synchronous in nature and return a type object casted according to your custom logic. In case no item exists in the cache, it returns null.
Look at the code below where a customer wants to see address details to make sure they are correct using the normal Get() method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// Pre-condition: Cache is already running // Items are already present in the cache // Initialize Cache ICache cache = CacheManager.GetCache("demoCache"); string customerKey = "Customer:ALFKI"; // Retrieve the Customer object Customer customer = cache.Get<Customer>(customerKey); if (customer != null) { Console.WriteLine($"Customer: {customer.ContactName}, Address : {customer.Address}"); } |
NCache also allows user to Add, Update, and Remove data from the cache.
NCache Details Get Operation Atomic Operations
Get Bulk items from Cache
Similarly, you can also fetch bulk items from the cache using the GetBulk() method. Using this, you can fetch numerous items from the cache using a single call.
The example below shows how multiple customers are fetched from the cache using a single operation.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
// Pre-condition: Items are already present in the cache // Create an array of all keys to fetch String[] keys = new String[] { "Customer:ALFKI", "Customer:ANATR", "Customer:ANTON", "Customer:AROUT", "Customer:BERGS" }; // Get items from cache IDictionary<string, Customer> retrievedItems = cache.GetBulk<Customer>(keys); // Retrieve customers and their addresses from dictionary foreach (KeyValuePair<string, Customer> retrievedItem in retrievedItems) { Console.WriteLine($"Customer: {retrievedItem.Value.ContactName}, Address : { retrievedItem.Value.Address}"); } |
Additionally, NCache provides the user to Add, Insert, and Remove bulk data in a single call.
NCache Details CRUD Operations Bulk Operation
Fetch Items using Keywords
You can also fetch items using keywords attached to them for an effective search. You mark your data with certain string-based keywords and retrieve the items with the help of these keywords. For example, you want to group certain customers using the region they belong to, such as East Coast or West Coast. The certain feature set provided by NCache for this purpose is:
Named Tags | Tags | Groups |
---|---|---|
Provided in the form of a dictionary | Provided in the form of an array of tags | Provided in the form of string |
Can be of any primitive data type | String based only | String based only |
In the example given below, the user wants to see details of all customers having 12% VIP membership discount using SQL query.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
// Pre-conditions: Items are already present in the cache with named tags // Custom class is query indexed through NCache Web Manager or config.ncconf // Create SQL Query with the specified criteria // Make sure to use the Fully Qualified Name for custom class string query = "SELECT CustomerID, ContactName FROM Alachisoft.NCache.Samples.Data.Customer WHERE VIP_Membership_Discount = 0.12"; // Use QueryCommand for query execution var queryCommand = new QueryCommand(query); // Executing Query ICacheReader reader = cache.SearchService.ExecuteReader(queryCommand); // Read results if result set is not empty if (reader.FieldCount > 0) { while (reader.Read()) { // Get the value of the result set string customerID = reader.GetValue<string>("CustomerID"); string customerName = reader.GetValue<string>("ContactName"); Console.WriteLine($"Customer '{customerName}' with ID '{customerID}' has VIP membership discount."); } } else { Console.WriteLine($"No VIP members found"); } |
For other operations using Named Tags, kindly refer to NCache Docs.
NCache Details ICacheReader Search Cache Data with Named Tags
Search Cache using SQL
For fetching items from a particular database, queries are an effective way to search with certain criteria. NCache fully understands the need for queries, letting you fetch items from the cache using SQL-like queries. Additionally, you need to index the particular item in the cache as it does not create indexes on its own, unlike the database. But you are good to go with SQL query once data is indexed.
It provides ADO.NET-compliant APIs like ExecuteReader, ExecuteScalar, and ExecuteNonQuery to search (SELECT) and remove (DELETE) data from the cache.
The following are the things supported in NCache queries:
- Basic query operators (=, ==, !=. LIKE, NOT LIKE)
- Logical operators (AND, OR, NOT)
- Aggregate Functions (SUM, COUNT, AVG, MIN, MAX)
- Miscellaneous
Look at the code below to see how SQL-like queries search through the cache.The following example shows a user who has applied the filter to show only those products having unit price less than 100.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
//Pre-condition: Items are already present in the cache // Use the Fully Qualified Name (FQN) of your own custom class string query = "SELECT * FROM Alachisoft.NCache.Samples.Data.Product WHERE UnitPrice < ?"; // Use QueryCommand for query execution var queryCommand = new QueryCommand(query); // Providing parameters for query queryCommand.Parameters.Add("UnitPrice", 100.0); // Executing QueryCommand through ICacheReader ICacheReader reader = cache.SearchService.ExecuteReader(queryCommand); // Read results if result set is not empty if (reader.FieldCount > 0) { while (reader.Read()) { // Get key of product item in cache string productKey = reader.GetValue<string>("$KEY$"); // Get product attributes string productName = reader.GetValue<string>("ProductName"); double unitPrice = reader.GetValue<double>("UnitPrice"); Console.WriteLine($"Product '{productName}' with key '{productKey}' has UnitPrice {unitPrice}"); } } else { Console.WriteLine($"No product found with price less than 100"); } |
NCache Details SQL Supported Operations SQL Query
Search Cache using LINQ
Like SQL, NCache provides support for writing queries in LINQ to search the cache. NCache LINQ provider converts LINQ-related queries into NCache’s extended SQL format and returns the results accordingly after transforming it into LINQ format. There are two types of syntax for writing LINQ queries:
- Lambda Expression
- Simple LINQ query expression
However, just like SQL, you need to define indexes for the searchable cache data for your query to run smoothly.
Look at the code example below to see how the LINQ query fetches an object from the cache. User has applied the filter to show either Beverages or Dairy Products having at least 10 units in stock.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
// Pre-condition: Items are already present in the cache // Create custom class LINQ object IQueryable<Product> products = new NCacheQuery<Product>(cache); // LINQ query to search cache IQueryable<Product> result = from prod in products where (prod.Category == "Beverages" || prod.Category == "Dairy") && prod.UnitsInStock > 10 select prod; if (result != null) { foreach (Product product in result) { Console.WriteLine($"Product '{product.ProductName}' in Category '{product.Category}' has stock of '{product.UnitsInStock}' units"); } } else { Console.WriteLine($"No product found"); } |
NCache Details LINQ Query LINQ Basic Operators
Concluding the Blog
NCache actually is a powerful caching solution for increasing the performance of read-intensive applications. It boosts speed and enhances performance, scalability, and reliability with a rich set of features. Please check out other NCache features from our website.