ASP.NET Core is becoming popular for developing web applications because of its cleaner and lighter architecture and cross-platform support. Such ASP.NET Core applications are high-traffic and run in a load-balanced multi-server deployment. In fact, it’s common to see 10-20 server web farms and a few even larger than this.
Having a multi-server load-balanced deployment makes your application tier very scalable because you can add more servers as your transaction load increases. This allows your ASP.NET Core application to handle heavy transaction loads easily. There is still a performance bottleneck that exists, and that slows down your ASP.NET Core application.
And this ASP.NET Core performance bottleneck is in your database and data storage that cannot handle heavy loads the way your ASP.NET Core application tier can. Although you can add more servers to the application-tier web farm, you cannot do the same with your database tier. Below are the two types of data storage that become a performance bottleneck for ASP.NET Core applications.
- Database Server (SQL Server)
- ASP.NET Core Sessions
NCache Details NCache Docs NCache Client API
The Solution: Distributed Cache
To remove these data storage performance bottlenecks, your best bet is to use a distributed cache like NCache. NCache is a .NET, open source in-memory distributed cache that is much faster than the database. Unlike your database, NCache is linearly scalable because it lets you build a cluster of cache servers and add more servers to the cluster as your transaction loads increase.
NCache lets you cache application data so you can reduce those expensive database trips by almost 80%. This reduces the load on the database which allows it to perform both reads and writes much faster and not become a performance bottleneck anymore.
NCache is also a scalable distributed store for your ASP.NET Core sessions. Additionally, NCache replicates ASP.NET Core sessions to multiple servers to prevent data loss in case any cache server goes down. For ASP.NET Core sessions, this is very important because you cannot afford to lose any sessions at run-time. Below is a diagram showing how a distributed cache like NCache fits into your application deployment.
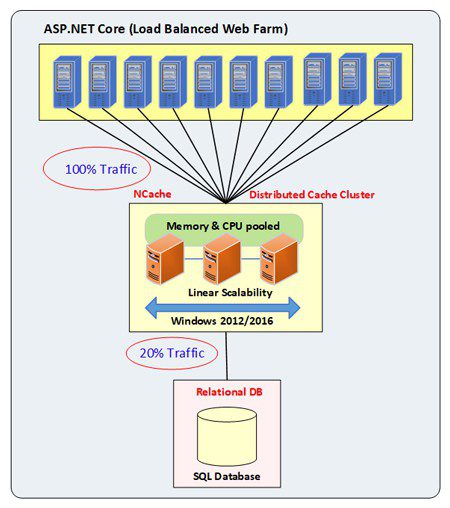
Figure 1: How NCache fits in your application deployment.
NCache Details NCache Docs NCache Client API
App Data Caching thru ASP.NET Core IDistributedCache
Before ASP.NET Core, the older ASP.NET provided a stand-alone ASP.NET Cache that didn’t meet the needs of multi-server environments. Now, ASP.NET Core has introduced the IDistributedCache interface as a fairly basic distributed caching standard API that lets you program against it and then plugs in third-party distributed caches seamlessly.
Here is an example of how to use IDistributedCache
interface:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
IDistributedCache _cache; ... private byte[] LoadCustomer(string custId) { string key = "Customers:CustomerID:" + custId; // is the customer in the cache? byte[] customer = _cache.Get(key); if (customer == null) { // the cache doesn't have it. so load from DB customer = LoadFromDB(key); // And, cache it for next time _cache.Set(key, customer); } return customer; } |
NCache has also implemented a provider for IDistributedCache
that you can plug in to your ASP.NET Core applications. This way, you don’t have to change any code specific to NCache.
Here is what the IDistributedCache
interface looks like (please note that each of these methods also has an Async overload).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
namespace Microsoft.Extensions.Caching.Distributed { public interface IDistributedCache { // Each of these methods also has an “Async” overload byte[] Get(string key); void Refresh(string key); void Remove(string key); // Specify absolute & sliding expiration through options void Set(string key, byte[] value, DistributedCacheEntryOptions options); } } |
Configuring NCache as IDistributedCache Provider
Here is how you configure NCache as your IDistributedCache
provider in your ASP.NET Core Startup
class.
1 2 3 4 5 6 7 8 9 10 11 |
public class Startup { ... public void ConfigureServices (IServiceCollection services) { ... services.AddNCacheDistributedCache(); ... } ... } |
NCache Details ASP.NET Core Caching ASP.NET Core Session Provider
Why Choose NCache API Over IDistributedCache?
If your caching needs are fairly basic and you want the flexibility of changing the distributed caching vendor seamlessly, then you should go ahead and use the IDistributedCache interface. It allows you to change your caching vendor seamlessly. But weigh that against the cost of not having a lot of advanced caching features.
Another option is to use NCache API directly from your ASP.NET Core application. NCache API is very similar to the legacy ASP.NET Cache API. It contains tons of features free of cost that enable you to take full advantage of an enterprise-grade distributed cache.
Remember, the more data you can cache, the greater the performance and scalability benefit to your application. And, without the advanced caching features, you’re often restricted to caching read-only or simple data. Read more about all the different NCache caching features that you miss by using the IDistributedCache
provider.
Storing ASP.NET Core Sessions in Distributed Cache
Prior to ASP.NET Core, the older ASP.NET provided an ASP.NET Session State Provider framework that allowed third-party session storage providers to plug-in. ASP.NET Core sessions provides a similar mechanism for plugging in third-party storage providers. Below are the two ways to use NCache as ASP.NET Core session storage:
Use NCache for ASP.NET Core Sessions thru IDistributedCache
As soon as you configure NCache as IDistributedCache
provider for ASP.NET Core, NCache automatically becomes the default storage option for ASP.NET Core sessions and you don’t have to do anything else. But please note that this implementation is limited in features as compared to the older (before ASP.NET Core) ASP.NET Session State.
Here are some of the things the default ASP.NET Core Sessions implementation lacks:
- Session locking: ASP.NET Core does not provide session locking. This is something even older ASP.NET Session State had provided.
byte[]
array for custom objects: ASP.NET Core forces you to convert all your custom objects into byte array before you can store is in the session. Even older ASP.NET Session State support custom objects.
Use NCache as ASP.NET Core Sessions Provider
To work around the default ASP.NET Core Sessions implementation through IDistributedCache
provider, NCache has implemented its own ASP.NET Core Sessions provider. This implementation has a lot more features than the default one.
Here is how you configure it in your Startup
class.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
public class Startup { ... public void Configure(IApplicationBuilder app, IHostingEnvironment env) { ... app.UseNCacheSession(); ... } ... } |
You can specify ASP.NET Core Session configurations for the above in appsettings.json
file as following:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
{ ... "NCacheSessions": { ... "CacheName": "demoCache", "EnableLogs": "True", "RequestTimeout": "90", "EnableDetailLogs": "False", "ExceptionsEnabled": "True", "WriteExceptionsToEventLog": "False" } ... } |
NCache Details NCache Docs Configure NCache IDistributedCache Provider
Conclusion
Microsoft provides two options as IDistributedCache
providers. One is SQL Server and second is Redis. NCache is better than both options. In comparison to SQL Server, NCache is much faster and more scalable. Also, NCache is also a better than Redis for the following reasons:
- Native .NET: NCache is 100% native .NET and therefore fits into your .NET application stack very nicely. On the other hand, Redis comes from a Linux background and is not a native .NET cache.
- Faster than Redis: NCache is actually faster than Redis as NCache Client Cache feature gives NCache a significant performance boost.
- More Features: NCache offers a number of very important distributed cache features that Redis does not. See more details in this Redis vs NCache.