NCache has lots of features: ASP.NET Cache provider and Full-Text searching, among others. But, this time, let’s see another NCache feature: Publisher/Subscriber (Pub/Sub) messaging. Let’s learn how to implement the Pub/Sub messaging pattern with NCache.
What’s Pub/Sub messaging?
Pub/Sub is a messaging pattern where senders (or publishers) share messages with multiple receivers (or subscribers) through a channel, avoiding coupling between senders and receivers.
With Pub/Sub messaging, we can share messages between applications and offload time-consuming operations to background processors. For example, in a Reservation Management System, we can resize room images in a background processor by “listening” to an event triggered by a Web application.
NCache provides an in-memory Pub/Sub messaging to enable real-time information sharing between .NET applications.
Thanks to distributed architecture, NCache offers a scalable, highly-available, and storage-efficient pub/sub messaging mechanism.
How to implement Pub/Sub messaging with NCache
Before moving to a sample application, let’s go through some terminology first.
With NCache, the channel to exchange messages is called a Topic. Each subscriber subscribes to a topic to receive the messages sent to it.
NCache provides multiple types of subscriptions between the subscribers and publishers. In Pub/Sub messaging, a subscription is the “interest” of one or more subscribers in a topic.
NCache has two types of subscriptions:
- Non-durable: With this subscription type, subscribers won’t receive any message sent while they are disconnected. This is the default subscription type.
- Durable: Subscribers won’t lose any messages while they’re disconnected. With Durable subscriptions, messages are stored until subscribers rejoin or the messages expire.
Also, NCache has these two types of policies:
- Shared: In this policy, a subscription could have more than one active subscriber at a time. If a subscriber leaves the network, the subscription keeps active. This policy is only supported by Durable subscriptions.
- Exclusive: Unlike the Shared policy, subscriptions with an Exclusive policy could have only one subscriber at a time. This policy is available for both Durable and Non-Durable subscriptions.
In Pub/Sub messaging, subscribers register to a topic by name. Later in our sample application, we will use this subscription method. But, NCache also supports a pattern-based subscription method. This means a subscriber listens
to multiple topics in a single call. For example, a subscriber listening to the pattern new-*
will receive messages sent to the new-movies
and new-series
topics.
For more details about topic priorities and messages parameters, check Pub/Sub Messaging Components and Usage.
With this terminology in place, let’s build a sample .NET application to notify movie fans about every new movie release. Let’s write two Console applications: one as a publisher and another as a subscriber.
1. Publish a New Movie Message
First, let’s create a Console app to publish some movies. Also, let’s install the Alachisoft.NCache.SDK
NuGet package, version 5.3.0.
In the Program.cs
file, let’s publish some random movies from IMDb to a newReleases
topic. Something like this,
Let’s go through it. First, we started by grabbing a reference to an NCache cache with GetCache()
. We’re using the default demoCache
created during the NCache installation.
Then, we created a new topic using CreateTopic()
with a topic name. This is the same name we will use later in the subscriber application. Also, we attached a callback to get notified of delivery failures.
To publish a message for every movie, we used PublishAsync()
with three parameters: an NCache Message
, the DeliveryOption.All
to notify all subscribers, and true
to get notified of failures.
The NCache Message
is a wrapper around the object to send. We should mark it as [Serializable]
. For example, here’s the Movie
record,
To build the Message
, we used the ToMessage()
extension method with an expiration.
If one of our messages expires and nobody receives it, the OnFailureMessageReceived
callback is called. For more details, check Messages Behavior and Properties.
For example, let’s run our Publisher application without any subscriber (yet) to let the messages expire,
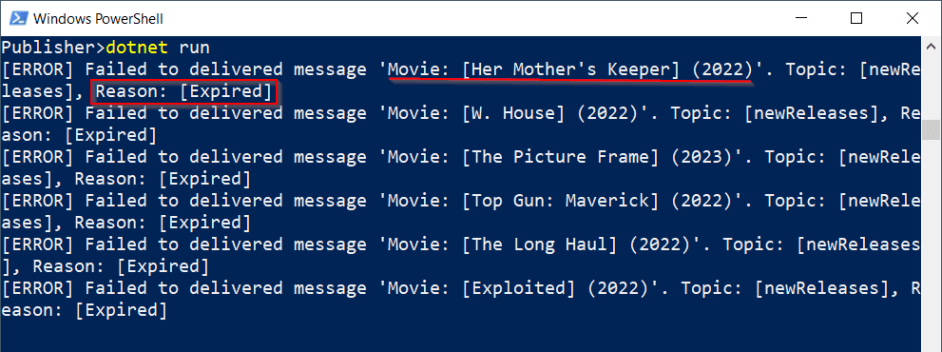
Figure 1: Run Publisher Application without any Subscriber
In our Publisher app, we used PublishAsync()
. Also, NCache has support to send multiple messages in bulk in a single call with the PublishBulk()
method.
The Publisher is ready. Let’s write the Subscriber application.
2. Subscribe to New Movie Messages
Let’s create another Console application to subscribe to the newReleases
topic.
The Program.cs
file looks like this,
This time, we referenced a topic one using the same topic name we used in the Publisher application and attached a callback in case of topic deletion.
Next, we create a non-durable subscription using CreateSubscription()
with a MessageReceived
callback and DeliveryMode.Async
. With this delivery mode, NCache doesn’t guarantee the order of messages. To receive messages in order, we should use DeliveryMode.Sync
.
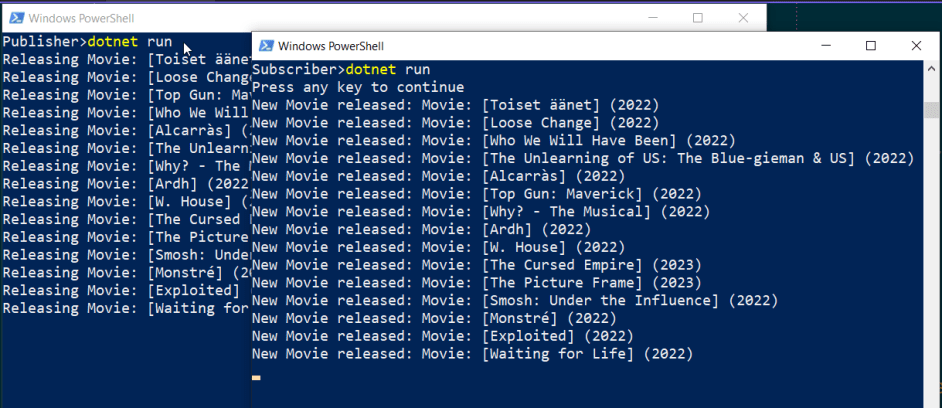
Publishing and subscribing to new movie releases