In high-transaction server environments, choosing the optimal communication channel for your applications is crucial. The Publisher/Subscriber (Pub/Sub) model is a popular choice for facilitating such communication. In this highly decoupled model, multiple clients publish messages as part of a message bus or intermediary communication channel, called a topic. Similarly, multiple clients can subscribe to these messages as part of this topic. This decoupling ensures that publishers do not send messages directly to subscribers but rather through the message bus, which handles all client communications.
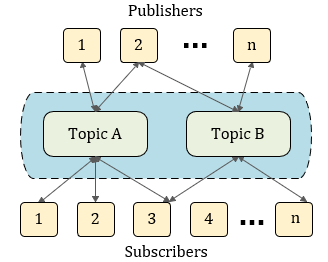
Figure 1: Publisher/Subscribe Model
In short, Pub/Sub messaging is a model where neither the publisher nor the subscriber knows each other’s identity. However, as the number of publishers and subscribers increases, the messaging load grows, potentially leading to scalability bottlenecks which defeat the primary purpose of a decoupled messaging interface. Let’s explore a distributed caching solution like NCache that retains the core benefits of Pub/Sub with the added advantage of improved performance and scalability.
Using NCache In-Memory PubSub
NCache provides fast, flexible, and highly scalable distributed caches for your .NET application. Using NCache as your event-driven Pub/Sub platform can be extremely beneficial for scaling your application seamlessly, preventing any system bottlenecks. Here’s how NCache acts as a messaging bus for your application:
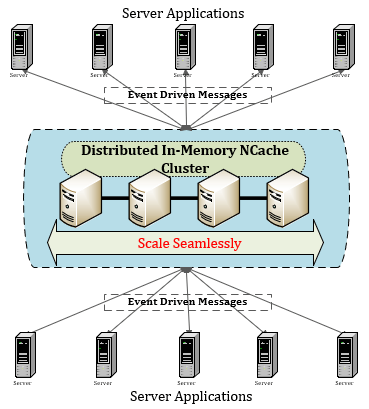
Figure 2: NCache as a Messaging Platform
NCache serves as an intermediary interface for your Pub/Sub setup, allowing multiple clients to subscribe to one or more topics simultaneously. Applications can connect to NCache servers and publish messages, which are then delivered to various clients based on their subscriptions. It lets you control whether messages are sent to all subscribers or just one, effectively acting as a messaging bus for your .NET server applications.
Quick PubSub Example with NCache
Imagine you have a global online gaming application where players interact through both audio and real-time text messages. As multiple players send messages to channels and teammates simultaneously, the volume of these messages can become enormous.
To handle this message load and ensure smooth communication, you need a robust messaging interface that enhances your game performance. For this purpose, NCache is the ideal choice for your game’s message bus. Here’s a code snippet demonstrating how server applications can publish and receive messages using NCache. This example shows how to obtain a topic and publish messages efficiently.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
// Precondition: Cache is already connected // Mention the name of the topic string topicName = "PLAYERMESSAGES"; // Create a Topic in NCache var topic = cache.MessagingService.CreateTopic(topicName); // Create and publish a message to the topic var message1 = new Message(new PlayerMessage { PlayerID = 1, MessageContent = "Ready for the next level!", Timestamp = DateTime.UtcNow }); PrintMessage(message1, "Publisher", null); // Publish the message with delivery option set to All subscribers topic.Publish(message1, DeliveryOption.All); |
Once a message is published against a topic, the subscribers create subscriptions against it to receive messages. The following code demonstrates how subscribing applications can register to a certain topic to receive desired messages.
1 2 |
// Register subscribers to this Topic var subscription1 = topic.CreateSubscription(MessageReceived_Subscription1); |
Types of Topic Subscriptions
NCache provides multiple types of topic subscriptions, i.e., Non-Durable, Durable (Shared and Exclusive), and Pattern based subscriptions as discussed below.
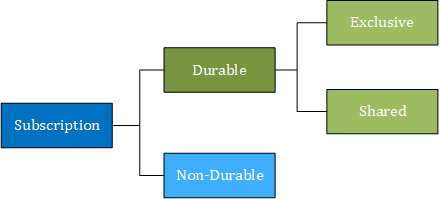
Figure 3: Types of Topic Subscriptions
Non-Durable Subscription
In a non-durable subscription, subscribers only receive messages while connected. If they disconnect, they miss any messages sent during this period. Such subscriptions are exclusive and automatically deleted upon disconnection. This means that if that subscriber rejoins or establishes the connection again, it will be considered a new subscription. For more information, refer to the NCache documentation.
Durable Subscriptions
In a durable subscription, the cache ensures that subscribers do not miss any messages even if they disconnect due to application or machine shutdown, restart, or network issues. Messages are stored on the server until the subscriber reconnects or they expire. Unlike non-durable subscriptions, durable ones are not automatically deleted on disconnection and remain active until unsubscribed.
- Exclusive Durable Subscriptions: An exclusive durable subscription allows only one active subscriber at a time. If the current subscriber unsubscribes gracefully, the exclusive subscription can be assigned to a new subscriber. If a subscriber leaves abruptly, the new subscription request is accepted after waiting for an idle time. The assigned messages always stay there even if there is no subscriber.
1 2 3 |
// Create and register subscribers for topic // Message received callback is specified IDurableTopicSubscription subscriber = topic.CreateDurableSubscription("RuntimePlayerComms", SubscriptionPolicy.Exclusive, MessageReceived, TimeSpan.FromMinutes(20)); |
- Shared Durable Subscriptions: Durability can also be achieved through shared subscriptions. In this type of subscription, more than one client can subscribe to a single subscription for load sharing. Messages are distributed using the Round Robin method to send messages to all connected clients. So, even if a client disconnects, the messages continue to be distributed among the remaining subscribers. Therefore, a subscription remains active as long as at least one client is connected.
1 2 3 |
// Create and register subscribers for the topic // Message received callback is specified IDurableTopicSubscription subscriber = topic.CreateDurableSubscription("RuntimePlayerComms", SubscriptionPolicy.Shared, MessageReceived, TimeSpan.FromMinutes(20)); |
Pattern Based Subscriptions
To streamline subscribing to multiple topics, NCache provides pattern-based subscriptions. This feature allows you to subscribe to all current and future topics that match a specified pattern. For more information on the supported wildcards in NCache’s pattern-based subscriptions, refer to the NCache documentation.
1 2 3 4 5 |
// Only ? * [] wildcards supported string topicName = "team*"; string subscriptionName = "TeamPlayersComms"; // Get the topic ITopic topic= cache.MessagingService.GetTopic(topicName, TopicSearchOptions.ByPattern); |
Conclusion
Why choose NCache? Here’s why:
- Speed: Being an in-memory solution, NCache delivers rapid performance.
- Scalability: It scales linearly by adding servers at runtime without disruption.
- Flexibility: It dynamically rebalances data, requiring no client intervention.
In essence, NCache offers a powerful, scalable, and fast messaging solution for your application. Its in-memory architecture effectively handles high message loads, making it an excellent choice for optimizing communication in your .NET application. Sign up and explore NCache’s messaging service today!