NHibernate is a popular object-relational mapping (ORM) solution for .NET applications that aims to simplify database programming. Therefore, many high-traffic applications use it. Unfortunately, these applications face database scalability bottlenecks. To tackle this issue, it provides its client applications with a caching infrastructure (an in-memory cache store) to prevent exhausting their databases with such a high request load. This type of caching includes first-level and second-level caches.
NHibernate First Level Cache and its Limitations
NHibernate First Level cache provides a basic standalone (InProc) cache associated with the session object. This cache is limited to current sessions only. By default, it reduces the number of SQL queries on the database per session.
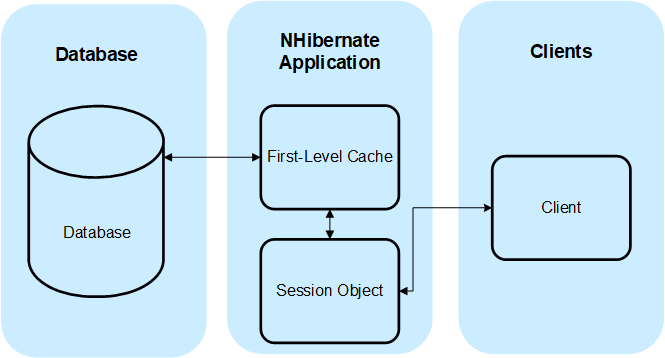
Figure 1: First-Level NHibernate Cache.
However, this does have some limitations, as follows:
- Each process has an individual first-level cache not synchronized with other caches, making data integrity a struggle.
- Cache size is limited to the process memory and cannot scale.
- Recycling worker processes cause the cache to be cleared, necessitating data reloads and reducing your application performance.
Solution: A Distributed Cache for NHibernate
The NHibernate Second Level Cache exists at the Session Factory level, which means multiple user sessions can access a shared cache. Moreover, it has a pluggable architecture, allowing applications to incorporate third-party distributed caches without code changes. Therefore, you can use the relevant NCache Provider as a Second Level Cache.
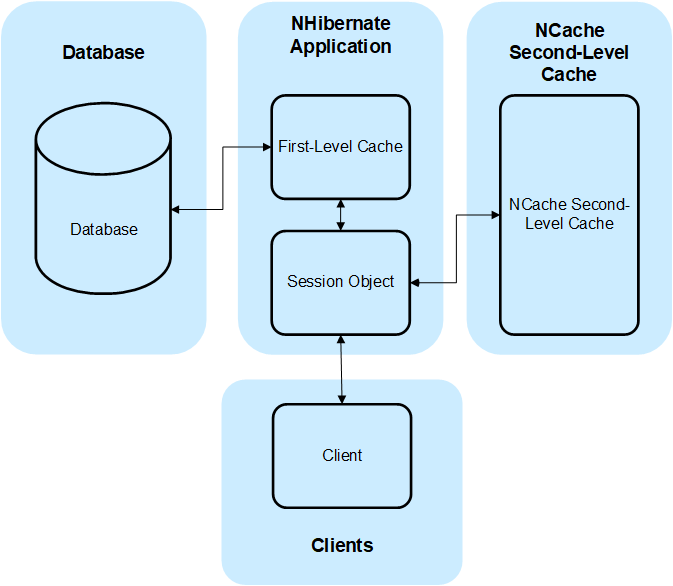
Figure 2: Second-Level NHibernate Cache.
You can use NCache in your NHibernate application in the following way:
Step 1: Add the following configuration in the Web.config under the <configSections> tag:
Step 2: Next, install NCache NuGet package for application without NCache installation using the following commands in Package Manager Console inside Visual Studio, based on your chosen installation, i.e., Enterprise, Professional, and Open Source:
Step 3: Configure NCache as NHibernate’s second-level cache provider by adding the cache.provider_class property under <hibernate-configuration>.
Step 4: Enable the use of a second-level cache in the application by setting the cache.use_second_level_cache property to True as shown below.
Step 5: Specify Application ID for NCache NHibernate Provider in NCacheNHibernate.xml.
Step 6: Next, you can configure cacheable objects as follows:
You can learn more about the tags used to configure cacheable objects here.
Advantages of NCache as a NHibernate Second Level Cache
Moreover, using NCache in this way has the following advantages:
- NCache synchronizes across multiple processes and servers, ensuring cache consistency and avoiding data integrity issues.
- Application process recycling does not affect the cache, as your application processes are stateless in an OutProc distributed cache.
- NCache pools the memory of all cache servers together, allowing users to add more servers to the cluster to increase cache size (by hundreds of gigabytes and even terabytes).
- Linear scalability to handle larger transaction loads as NCache scales linearly and reduces your database traffic by as much as 90%.
- NCache provides support for InProc Client Caches, i.e., a cache-on-top-of-a-cache running within your worker process, letting you boost performance within your applications.
Conclusion
Using distributed caches like NCache as NHibernate second-level caches enables you to use NHibernate and run your application in a multi-server configuration, allowing you scale your application and handle high transaction loads. Download and sign up for a fully working 30-day trial of NCache Enterprise and try it out for yourself.